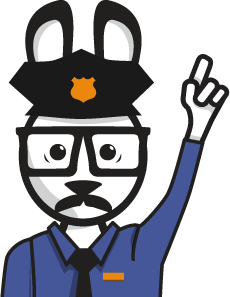
Numbers are an essential part of any language including JavaScript.
We have already covered that JavaScript has three data types: Strings, Numbers, and Booleans. It only has one variable to store all three data types; it’s up to you to look at this as a pro or a con. It’s a good idea to make peace with it and treat it as a pro; you know, the glass half-full approach.
Moving back to numbers, we can kill two birds with one stone. First let’s see what PROMPT is in JavaScript. "User input" is when you ask the user for data. How exactly will the data come to us so we can access it in our code and manipulate it? "prompt" is the answer for now(in a real live application if it was 1987 but for now would be the correct answer as well). prompt() is a function which permits us to get data from the user: watch the video carefully and you will see how it provides this interface.
prompt() accepts two string parameters. The first one is the message which will be displayed on the pop-up box like “How Old Are You?” while the second argument is what should be displayed in the text box where the user will input the data. This argument is usually left blank unless you want users to have a certain default string so they can just click OK.
What will "prompt" return to us? Whatever the user enters in the prompt box will be returned as a string. Remember "prompt" only returns a string, even if the user enters 23. "23" will be stored in a a string and cannot be used as a number. Here it is in action:
var userAge = prompt(“Enter your age”, “ ”);
Let’s look at this string returning in detail. We have a variable var num1 which has 10 stored in it: we will ask the user for a number which we can add to variable num1. We use "prompt" and ask the user for the second number, a pop up box is displayed with the message “Please Enter a Number”: the user types 10, which we store in another variable called var num2. Now we display the result using "alert" like this:
alert(num1 + num2);
What do you think we will get?
If you answered 20 -- BEEP. Your logic is right but the answer is wrong, JavaScript does not know that num1 and num2 are Numbers, remember? It assumes they are strings and simply concatenates num1 and num2. The alert will give us “1010” rather than 20.
How do we solve this? JavaScript provides us with numerous functions. You just have to learn to choose the right function at the right time. In this case we want to add num1 and num2 -- we use the function parseFloat(); it accepts a string argument and outputs a number. Simple as that.
Now when we call "alert" like this:
alert(parsetFloat(num1) + parseFloat(num2));
We will get the desired result of 20, because parseFloat(); converts our string inputs into what JavaScript now recognizes as numbers: therefore it adds them instead of concatenating the strings.
ParseFloat(); can also convert to decimal values. If we want an integer there is parseInt(); which returns only integer values. We can additionally perform all the basic math functions on variables such as num1/num2, num1*num2, num1-num2. Play around with this and you'll quickly learn it.
It’s a good idea to watch this video more than once as it will help you understand how JavaScript deals with numbers and converts them from strings.
Have a question but don't want to ask it publicly? If you are a prime member just ask right here and we will get back to you within 48 hours. Not prime yet? no worries you can ask publicly below.